Array Find from Last
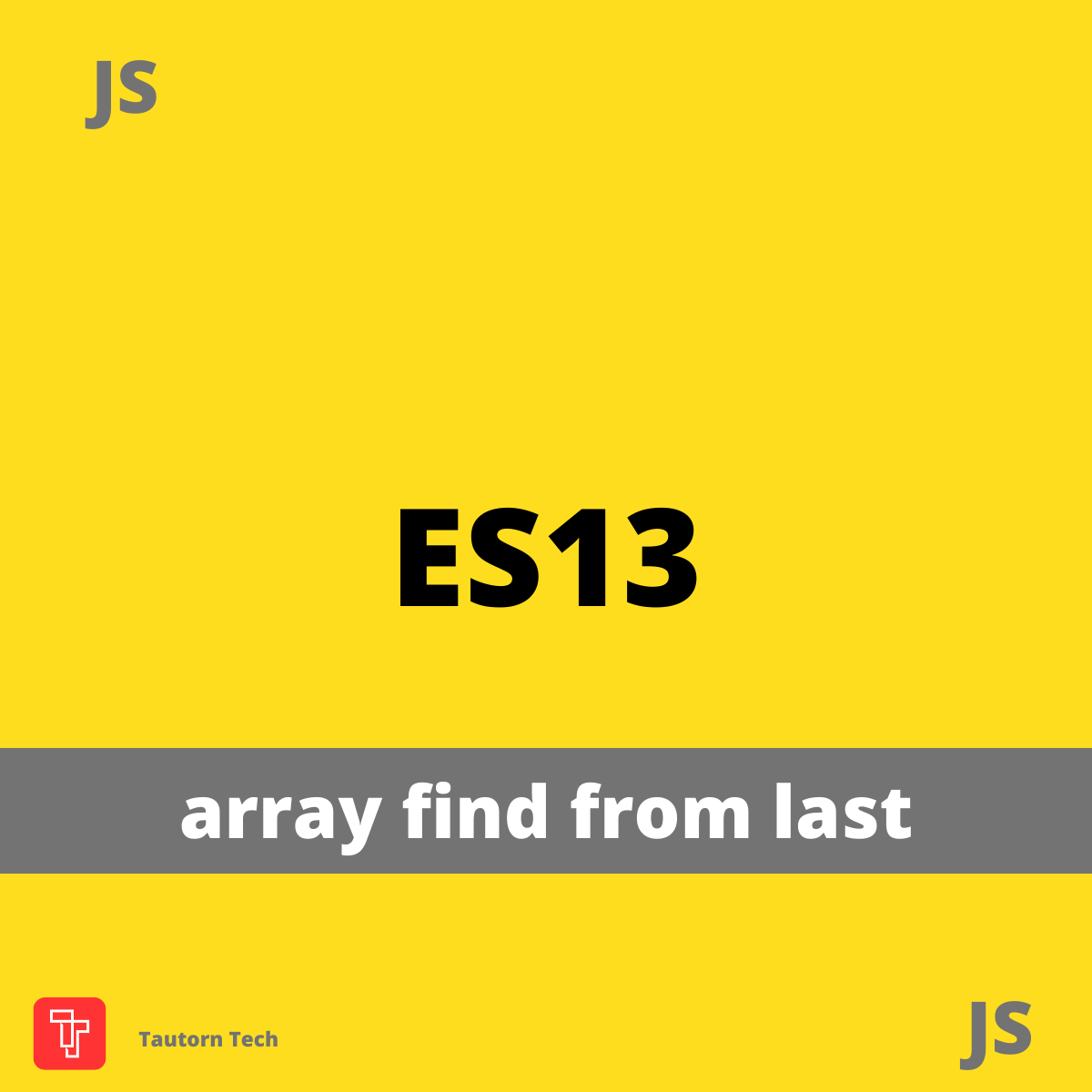
Motivation
Proposal to add Array.prototype.findLast
and Array.prototype.findLastIndex
to the ECMAScript standard library.
Array.prototype.find
is a very useful method, but it only finds the first element that matches the predicate. Sometimes, we want to find the last element that matches the predicate. This is currently not possible without iterating over the entire array.
A common pattern to find the last element is to reverse the array and then use find
method. Like this:
const array = [{ id: 1 }, { id: 2 }, { id: 3 }, { id: 4 }, { id: 5 }]
const matchFn = (element) => element.id % 2 === 0
const lastElement = [...array].reverse().find(matchFn)
// { id: 4 }
const lastIndex = [...array].reverse().findIndex(matchFn)
// 3
That approach is not very efficient, because it creates a copy of the array and then iterates over the entire array. Semantic is an issue too.
lastIndex
too has a problem because to get original index, we need to subtract the index from the length of the array.
const array = [{ id: 1 }, { id: 2 }, { id: 3 }, { id: 4 }, { id: 5 }]
const matchFn = (element) => element.id % 2 === 0
const lastIndex = [...array].reverse().findIndex(matchFn)
// 3
const originalIndex = array.length - lastIndex - 1
// 1
Proposed solution
This proposal adds findLast
and findLastIndex
methods to the standard library.
const array = [{ id: 1 }, { id: 2 }, { id: 3 }, { id: 4 }, { id: 5 }]
const matchFn = (element) => element.id % 2 === 0
const lastElement = array.findLast(matchFn)
// { id: 4 }
const lastIndex = array.findLastIndex(matchFn)
// 3
This code is shorter and more efficient than the previous one, more readable too.
Status
This proposal is currently at Stage 4.
Full documentation can be found here.